Being a software developer can become really interesting and fascinating.
After a few years as an employee, I decided to go on my own to be able to explore more, get out of my comfort zone ( I’ll tell you later why this is bolded) and have more time for my side projects.
As a freelancer, you’ll find all kinds of interesting projects, from the most stupid ideas to the ones that will challenge you in a good way.
Fortunately, my first project as a freelance software developer was from the second category. It required building an Android library that would run some Go files.
Well, at first, I wasn’t so happy about this. After a few years working only with React, React-Native, Elixir, PHP, NodeJs, and Angular, this was something new for me. But, that’s why I decided to become a freelancer and I took this challenge.
When you struggle with a problem, that’s when you understand it — Elon Musk
In this article, we’ll build a Go file as an Android app library (.aar) and import it in an Android application.
To accomplish the project requirements, It was necessary to use Gomobile which is a tool for building and running mobile apps written in Go.
Adding the Gomobile package is very simple:
$ go get golang.org/x/mobile/cmd/gomobile
After running the command from above, test if everything is ok with the following command:
$ gomobile
You should be able to see the following output:
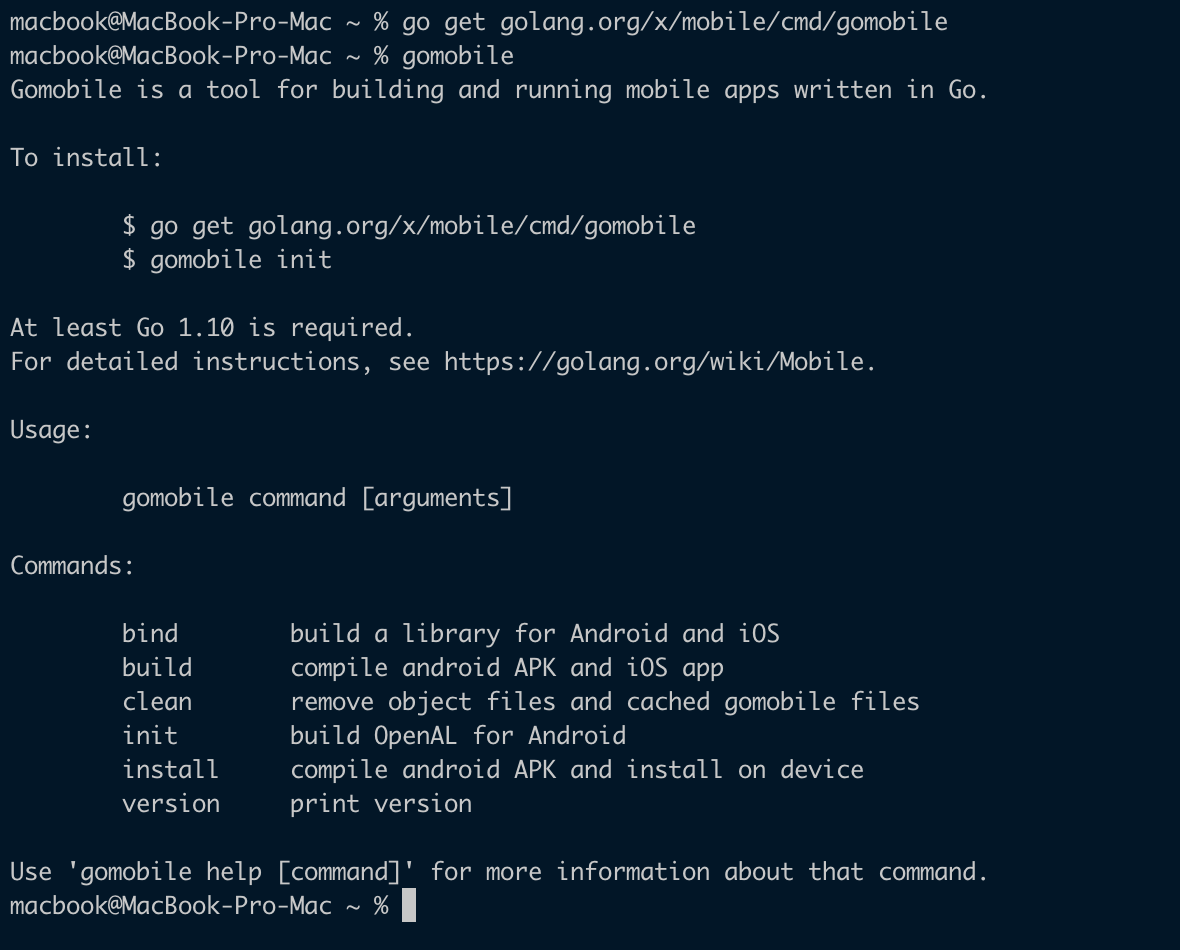
Great, the first step is accomplished. You can see that gomobile
has many useful commands, but we will use just bind
command.
Now, let’s write some code.
Create a new folder in your Go workspace. I am using github.com/andreirat
as my base path. You can create a new folder from the command line:
$ mkdir -p $GOPATH/src/github.com/your_github_username/hello
Let’s create a file inside that folder.
$ nano $GOPATH/src/github.com/your_github_username/hello/hello.go
Inside the hello.go
file, add the following code:
package hello
import "fmt"
func SayHello() string {
return "Hello!"
};
func SayHi(name string) string {
return fmt.Sprintf("Hi, %s!", name)
}
Now, let’s create an Android app that will use our Go file. For this, we need Android Studio.
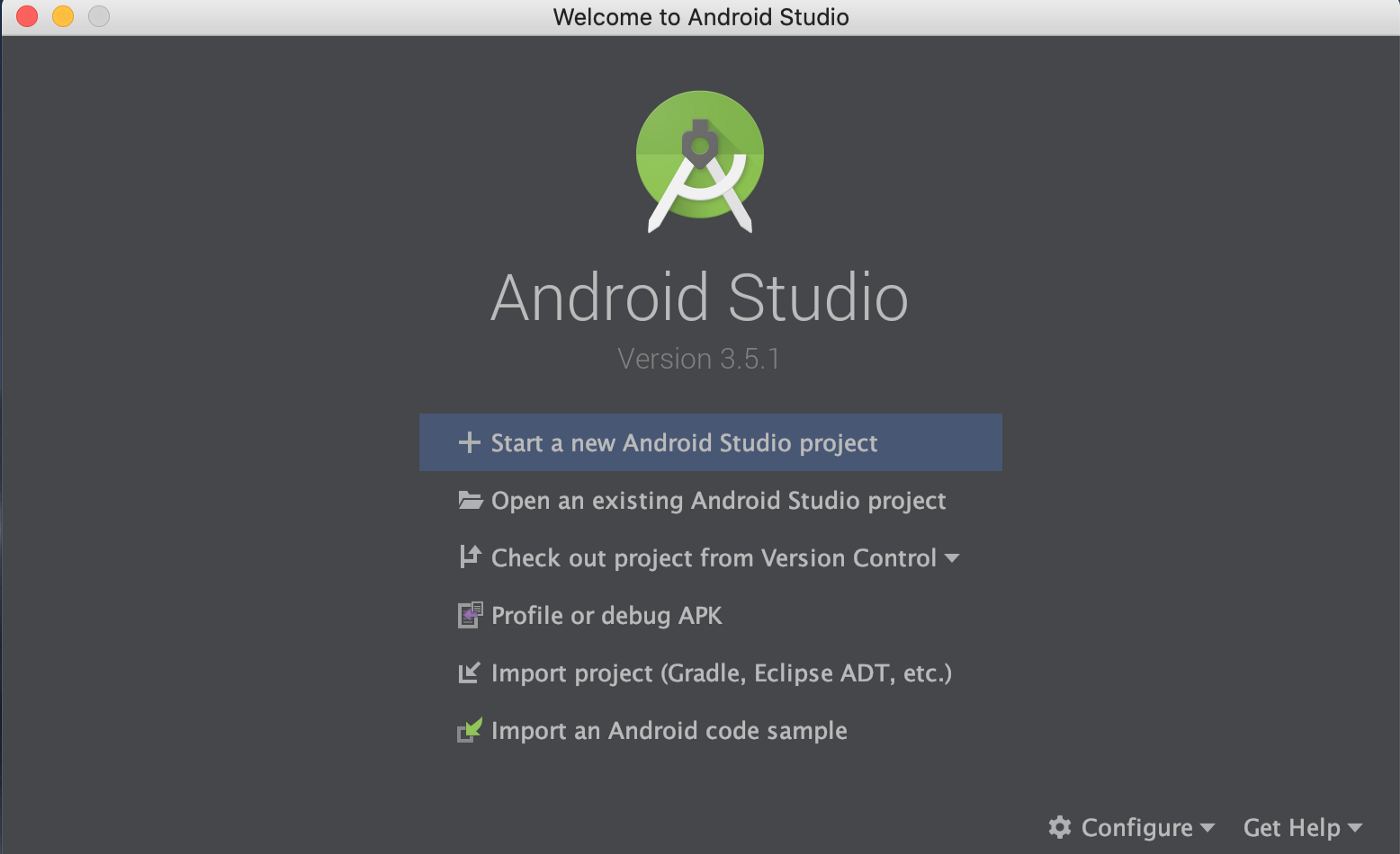
Click on Start a new Android Studio project

Select Fragment + ViewModel. You can choose any other activity, it works the same.
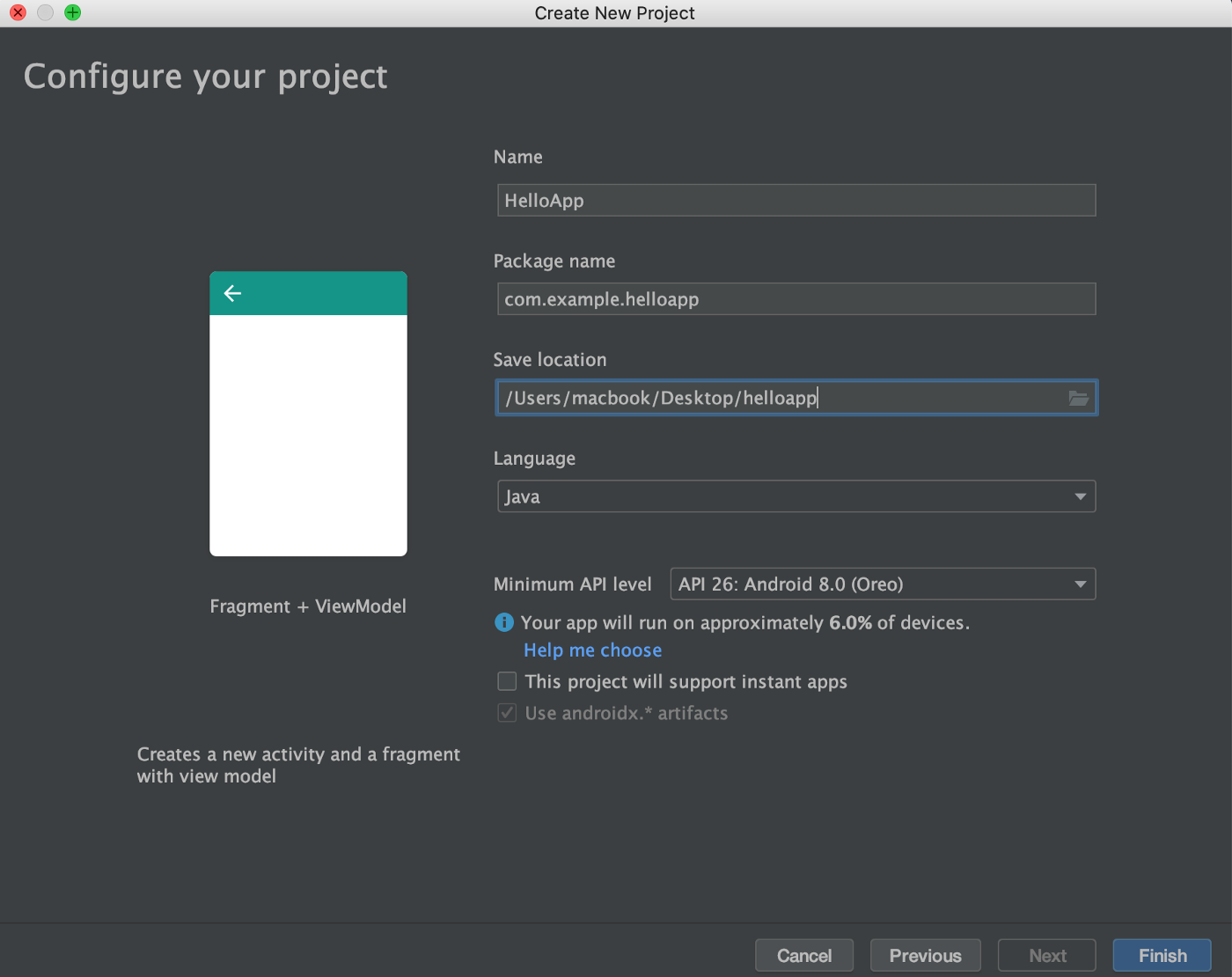
Give it a name and select the language. I am doing this in Java, but it works the same with Kotlin.
Now that the Android application is created, the next step is using the gomobile bind
command to create the .aar
file.
Open a terminal and navigate to your Android application root folder. From there, run the following command.
$ gomobile bind -o app/libs/hello.aar github.com/your_github_username/hello
This command will create hello.aar
file inside /app/libs/
.
In Android Studio, switch to the project view.
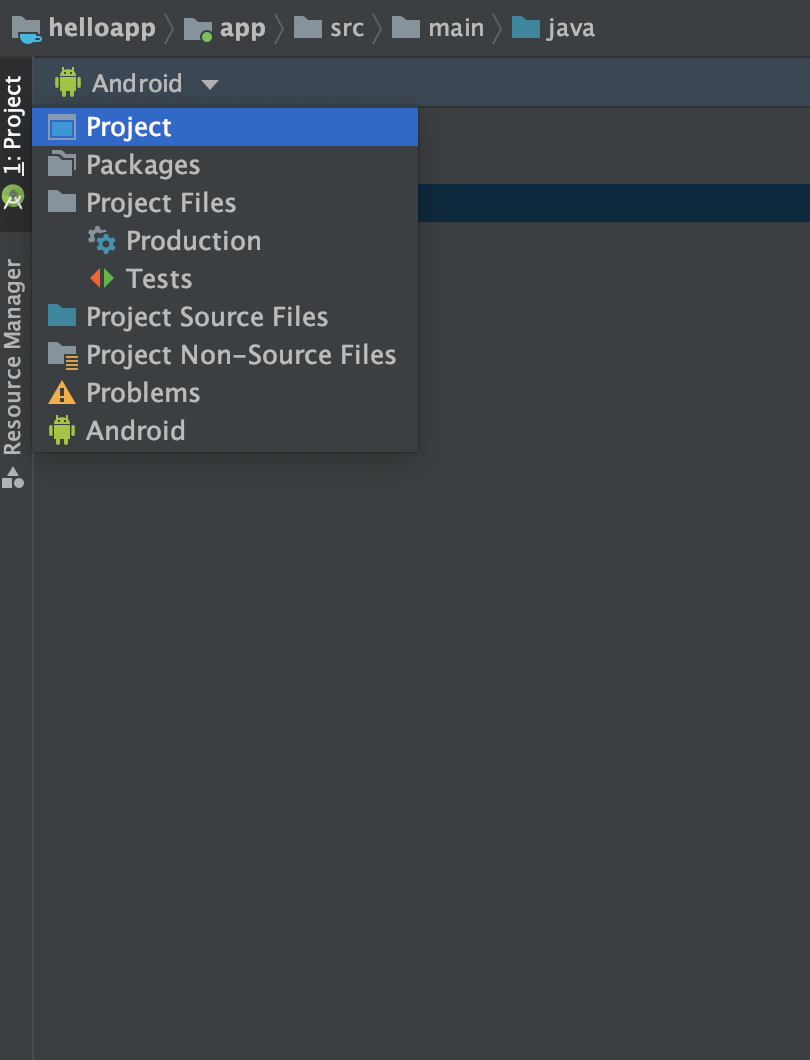
Navigate to /app/libs
and there you will find the Android app library recently created by the gomobile tool.

Now that the file it’s created, it needs to be imported. Open the file /app/build.gradle
and add the following line to your dependencies:
implementation (name:'hello', ext:'aar')
Inside the same file, add the following code. Android Studio needs to know where to find the file, in our case inside the /libs
folder.
repositories {
flatDir {
dirs 'libs'
}
}
Your gradle.build
file should look like this:

Click on Sync Now and the library should be imported and ready to use. The next step is to call the methods written in Go.
Open MainFragment.java
and change the function onCreateView
.
@Nullable
@Override
public View onCreateView(@NonNull LayoutInflater inflater, @Nullable ViewGroup container,
@Nullable Bundle savedInstanceState) {
View root = inflater.inflate(R.layout.main_fragment, container, false);
final TextView textView = root.findViewById(R.id.main_text);
mViewModel = ViewModelProviders.of(this).get(MainViewModel.class);
mViewModel.getText().observe(this, new Observer<String>() {
@Override
public void onChanged(@Nullable String s) {
textView.setText(s);
}
});
return root;
}
Next, open MainViewModel.java
modify the code as follows:
package com.example.helloapp.ui.main;
import androidx.lifecycle.ViewModel;
import androidx.lifecycle.LiveData;
import androidx.lifecycle.MutableLiveData;
// Import the library
import hello.Hello;
public class MainViewModel extends ViewModel {
private MutableLiveData<String> mText;
public MainViewModel() {
mText = new MutableLiveData<>();
// Call SayHello function from Go file.
String message = Hello.sayHello();
// Call SayHi function from Go file.
String hiMessage = Hello.sayHi("Andrei");
// Return hiMessage or message to try both methods.
mText.setValue(hiMessage);
}
public LiveData<String> getText() {
return mText;
}
}
Run the app. Our message is displayed on the screen.
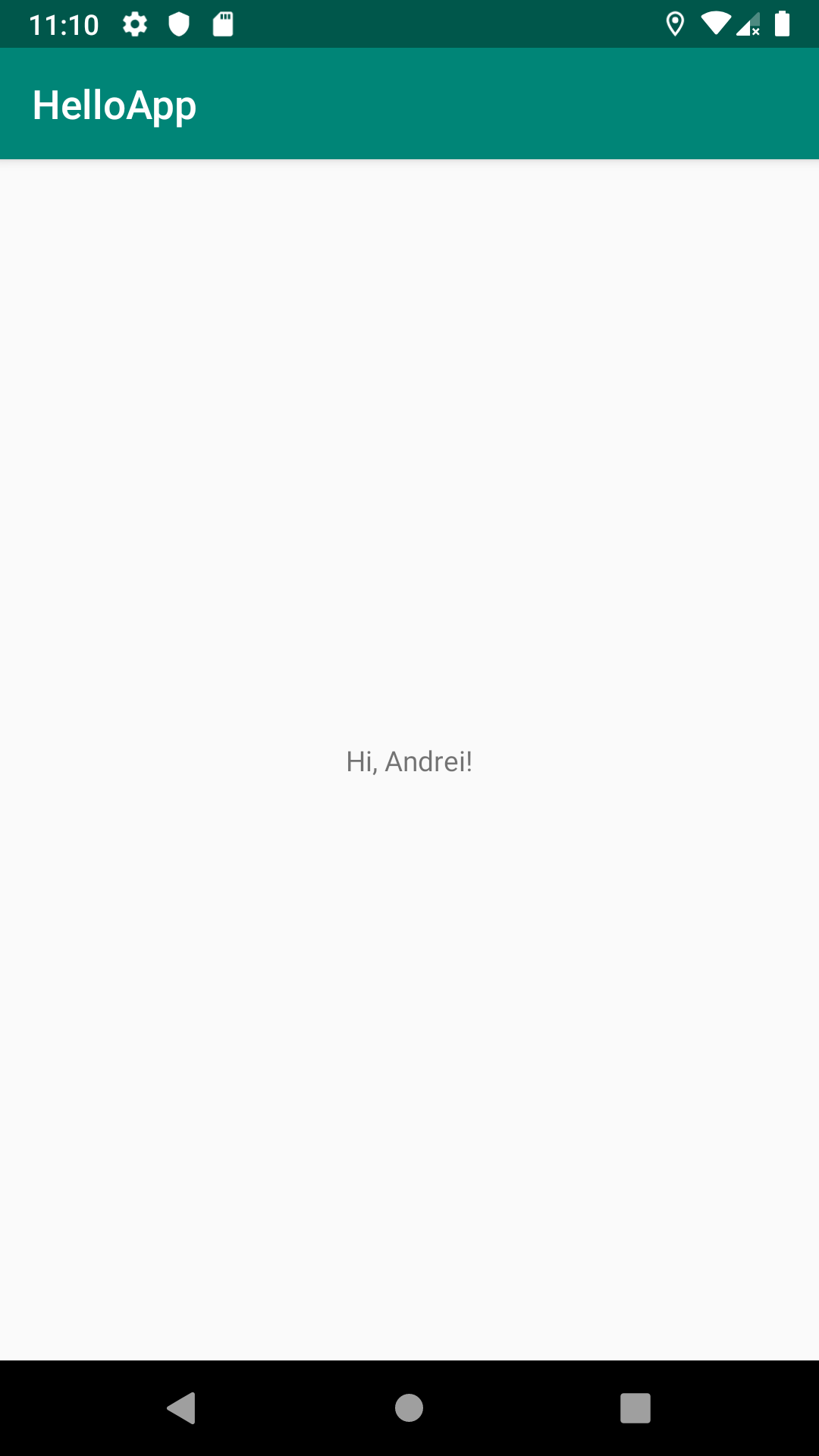
Awesome, now we have an Android app that prints a message from Go. This is just a basic example that can be developed more. Feel free to explore.
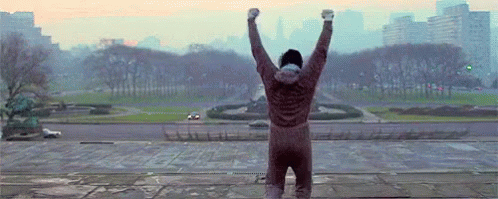
Remember the bolded phrase from the beginning of this article? Well, it’s bolded because exploring more and getting out of the comfort zone is required to help you not only in your personal development but also in your career, especially if you are a freelance software developer. Also, it will help you bring more clients.
Explore more, find what you like and always return something to the community.
If you have any questions regarding this article, you can find me on LinkedIn or Instagram.
Member discussion